Python Objects
The official Python documentation describes data on Python as follows:
Objects are Python’s abstraction for data. All data in a Python program is represented by objects or by relations between objects.
(Data Model – Python 3.8 Documentation)
What is an object?

In Python, an object is a fundamental concept that represents a piece of data and the operations that can be performed on that data. Everything in Python is an object, and each object has a type.
For example, numbers, strings, lists, and even functions are all objects. Think of objects as containers that hold data and come with built-in functions, or methods, that you can use to interact with that data.
So, when you’re working with Python, you’re essentially creating, manipulating, and using objects to perform various tasks. Objects make Python a versatile and object-oriented language!
Properties of Object
To make it even more relatable, let’s think about objects as “single persons”.
Imagine an object as an individual person. Now, every person has characteristics or attributes that make them unique—let’s call those attributes “properties” in the context of objects.
Additionally, person can perform actions or behaviors—walking, talking, eating. In the object world, we call these actions; “methods.”
Property
Person has attribute information such as “name,” “age,” “gender,” and “address. Each Python object also has such attribute information. The attribute information of an object is called a property.
Methods
Person behaves (acts) in such ways as “eating,” “sleeping,” and “talking.
Each Python object has such behavior. The behavior of an object is a method.
When you create a new object in Python, it’s like bringing a new person into existence. You define their properties, and they come with methods that you can use.
So, in a nutshell, objects in Python are like individuals with unique characteristics (properties) and abilities (methods), making your code more intuitive and human-like!
Difference between a method and a function.

A “method” of an object corresponds to an instruction that can be executed in Python.
A “function” is also an instruction.
So what is the difference between a “function” and a “method”?
“Functions” such as print() and input() were to be written as follows
Function name (argument)
In Python, when you’re working with functions, all you have to do is specify the function name. It’s pretty straightforward—you just call the function, and it does its thing.
In contrast, a “method” is described as follows
Data. Method name (argument)
Now, when it comes to methods, it’s a bit different.
Think of methods as special functions that are closely tied to specific objects. To use a method, you need to connect it to the object it belongs to by using the dot (.) notation. So, it’s like saying, “Execute the method XXX that belongs to the object XXX.”
To make it clearer, consider the dot (.) as a connector, like the word “of.” It links the data (object) with the method name, creating a relationship between them. In simpler terms, the dot (.) helps you say, “Execute the __ of △ △ △,” where the blank is filled with the specific method tied to the object △ △ △.
In the upcoming section, let’s put the “method” directive into practice.
String Methods

All data in Python is an object, so both numbers and strings are objects and have methods. Many of the methods that numerical objects (numeric types) have are related to mathematical expertise, so they will be omitted from this curriculum. If you are interested, please refer to the official documentation below.
Compared to numerical values, the methods of string objects (string type) are relatively easy to handle, so we will pick up a few methods from the following document.
Convert characters to uppercase or lowercase
Use the lower() method to convert charactors to lowercase, and the upper() method to uppercase.
Let’s check with the Jupyter Notebook.
a = "Hellow World"
a.lower()
output:
hellow world
a.upper()
output:
HELLOW WORLD
By converting it to either lowercase or uppercase using the methods lower() and upper(), you can manipulate the text stored in a variable.
When you apply these methods to the string data, like “Hellow World” in example above, a new modified string is generated.
However, it’s important to understand that this modification doesn’t automatically update the original content stored in variable “a.”
To actually overwrite the data in “a”, you should use an assignment statement. For example, if you want the text in “a” to be in lowercase, you would write following;
a = a.lower()
Similarly, if you prefer uppercase;
a = a.upper()
This assignment ensures that the changes made by lower() or upper() are reflected in the actual data held by the variable “a.”
Methods that preserve the existing data and do not directly modify it when invoked are referred to as “nondestructive methods”.
Search Strings
To locate a particular set of characters within a string, you can use the find() method in Python. Simply provide the string you’re searching for as an input to the find() method, as demonstrated below.
a = "Hellow World"
a.find("o")
output:
4
a = "Hellow World"
a.find("a")
output:
-1
When you use this method, it tells you the position (given as an integer, like “Xth”) of the first occurrence of the character you’re searching for. It’s important to know that counting starts from 0, not 1.
Searching for “a” returns -1 because there’s no “a” in “Hellow World.” If the character you’re searching for isn’t found, the method returns -1 as well.
Counting Strings
If you want to know how many strings are included, you can use count().
a = "Hellow World"
a.count("o")
output:
2
Output is 2 because there are two o’s. If there are none, it returns 0.
String Replacement
In Python, if you want to substitute one string with another, you can employ the replace() method.
This method takes two arguments separated by a comma: on the left side is the search string, and on the right side is the string you want to replace it with.
a = "Today is a sunny day."
a.replace("sunny", "rainy")
Output:
Today is a rainy day.
The above example replaces “sunny” with “rainy”.
However note that, just like with any other nondestructive methods, the original string remains unchanged unless you assign the result back to a variable.
To apply the replacement and update the content, you need to specify a variable and assign the result of the replace() method to it.
a = "Today is a sunny day."
a = a.replace("sunny", "rainy")
This ensures that the changes made by the replace() method are reflected in the actual data stored in your variable.
Trim Strings
The process of removing extraneous characters (such as whitespace and newlines) is called “trimming”, and Python strings have a convenient strip() method that does the trimming for you.
a = " Hellow World "
print(a)
a = a.strip()
print(a)
Output
Hellow World
Hellow World
No argument is required, but a specific string to be trimmed can be specified as an argument.
a = "#####Hellow World#####"
a = a.strip("#")
print(a)
Output
Hellow World
The return value is the trimmed string, but this is also a nondestructive method.
Format() Method

In Python programming, mastering string manipulation is an essential skill. One powerful technique is embedding the contents of a variable into a string.
This is achieved through the versatile format()
method, which allows you to create dynamic and personalized strings tailored to your specific needs.
We’ll take a closer look at the concept of embedding variables in strings using the format()
method and explore the various ways it can enhance your Python coding experience.
When you need to combine the content of a string with a variable in Python, you can use the format()
method as an alternative to simply separating them with a comma within the print()
function.
This method provides a more versatile and structured way to merge strings and variables. Here’s a simple example to illustrate the difference:
# Traditional concatenation using comma
name = "Alice"
age = 30
print("Hello, " + name + "! You are", age, "years old.")
# Using format()
print("Hello, {}! You are {} years old.".format(name, age))
In the first example, we concatenate strings and variables with the “+
” operator and commas within the print()
function.
In the second example, we use the format()
method to embed variables into the string by replacing {}
placeholders with the actual values of the variables. The format()
method provides a cleaner and more flexible approach, especially when dealing with multiple variables or complex string constructions. It’s a handy tool to have in your Python toolkit for creating more readable and dynamic output.
When you find using “format()” a bit cumbersome, there’s a more concise way to embed variables into strings in Python. You can directly place the variable names inside curly braces {}
within the string and add an “f” prefix before the opening quotation mark. This approach, known as f-strings, simplifies the process of incorporating variables into strings, and it doesn’t require worrying about the order of variables. Here’s a straightforward example:
# Traditional format() method
name = "Bob"
age = 25
print("Hello, {}! You are {} years old.".format(name, age))
# Using f-string for simplicity
print(f"Hello, {name}! You are {age} years old.")
In both cases, we achieve the same result of embedding variables into the string. However, the f-string method with the “f” prefix is more concise and often considered more readable. This can be particularly helpful when dealing with complex strings or when you want to enhance the clarity of your code. So, if you find yourself writing “format()” frequently, give f-strings a try for a more streamlined approach to string formatting in Python.
Summary.
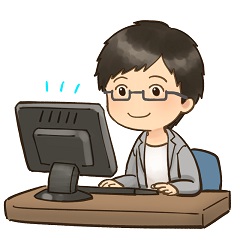
You have learned about “object” and “methods.” There are many different types of string methods alone. As with functions, it is difficult to learn all of them at once. It is better to learn them mainly by focusing on the ones you use frequently.