In this article, we’ll dell with concepts of “conditional branching,” “iterations,” and “exception handling.” Unlike simple sequential processing, these processes are essential tools that will play a pivotal role in expanding your programming capabilities. Get ready to create more sophisticated and dynamic programs.
In programming languages including Python, programs are basically executed from the top to bottom. For example, suppose you write the following:
print("Hello")
print("Python")
print("world!")
Output:
Hello
Python
world!
This is called “sequential processing“.
Conditional Branching

Sometimes, when you want to make your program do different things based on specific conditions or repeat the same action multiple times, you need to write your code in a slightly different way. This concept is known as “conditional branching.”
Conditional branching allows your program to make decisions.
For example, you can instruct the program to take one path if a certain condition is true and another path if it’s false.
In this article, you’ll discover how to write a program that can handle such situations using conditional branching.
“if statement”
Consider a program that displays “Old Enough!” if the test score is 21 or higher.
In this kind of program, the process changes depending on whether age is above or below 21. Check the flowchart below, the processing is divided at the ◇ point.

- If age is 21 or older, “Old Enough!” is displayed.
- If the age is less than 21, “Old Enough!” part is skipped and the next process, “DONE”, is displayed.
When describing a process that branches according to conditions, an “if statement” is used.
age = int(input("Enter Your Age:"))
if age >= 21:
print("Old Enough!")
print("DONE")
The input() is a function to pause processing and ask for data-input into the displayed text box. Afer data-input, it allows to restart processing.
Note that all data received by input() is the “string” type, so it is converted to a numerical value by int().
When score = 40 :
Old Enough!
DONE
When score = 10 :
DONE
In this code, the line “if age >= 21:” is like a decision point in Python.
It’s called an “if statement”, and it helps the program make choices based on conditions. The “if” keyword means “if,” and whatever comes after it is the condition we’re checking.
So, when we say “if”, it’s like asking a question. If the answer to that question is “yes” (meaning the statement after “if” is true), then the indented code block below it will be executed. The colon (:) shows where the condition ends.
In this specific code, the condition is “age >= 21,” which means “Is age greater than or equal to 21?” If the answer is yes, the program will print “Old Enough!” Otherwise, it will move on to the next part of the code without printing that message.
indentation of “if statement”
Modify the previous code as follows;
age = int(input("Enter Your Age:"))
if age >= 21:if age >= 21:
print("Old Enough!")
print("Let's go out to drink!")
print("DONE")
When age = 25 :
Old Enough!
Let's go out to drink!
DONE
In this time, if someone’s age is 21 or older, the program will show two sentences;
“You have passed”
and
“Let’s go out to drink!”
Because these two lines are indented.
In Python, it’s recommended to use four single-byte spaces for indentation. This indentation is like a way of grouping lines together. So, when the condition is met, the program knows to execute both indented lines as a group.
This indentation enhances code readability and shows the scope of control structures.
Specifically, when you indent instructions below a line like “if age >= 21:”, those indented instructions are the ones that will be executed conditionally—meaning they will only happen if the condition “age >= 21” is true.
So, proper indentation is crucial for Python to understand which code belongs to the conditional branch.

At first, you may be confused by the use of indentation to indicate the range of applicability, but you will gradually get used to it.
Conditional Expression with Logical Operator
Logical operators such as “and” and “or” can also be used in conditional expressions.
height = int(input("Enter Your Height:"))
if (height >= 5) and (heith <= 6):
print("It fits perfect")
print("DONE")

When height = 5.2 :
It fits perfect
DONE
When height = 4.5 :
DONE
Nesting: “if statement” within “if statement”
Another way to specify multiple conditions is to a technique called “nesting“, which involves putting an “if statement” inside another “if statement”.
This allows you to consider different scenarios within each other. It’s like having a decision inside another decision, helping you create more complex conditions in your code.
height = int(input("Enter Your Height:"))
if height >= 5:
if heith <= 6:
print("It fits perfect")
print("DONE")
When height = 5.2 :
It fits perfect
DONE
When height = 4.5 :
DONE
When you check if someone’s height is both greater than or equal to 5 and less than or equal to 6, you can use ‘and’ or nested “if statement”. The ‘step-by-step’ process is similar, but nesting can make it clearer. To show the inner process, you double the indentation, adding 8 spaces when describing what happens inside the inner “if statement”.

if-else statement
For example, if you want to display “Old Enough!” for 21 or older, but “Too Young” for less than 21, you can use an if-else statement to indicate that the condition is not met.

In that case, add the “else” to make it an “if-else statement”.
age = int(input("Enter Your Age:"))
if age >= 21:
print("Old Enough!")
print("Let's go out to drink!")
else:
print("Too Young")
print("DONE")
When age = 30 :
Old Enough!
Let's go out to drink!
DONE
When age = 18 :
Too Young
DONE
When the conditional expression is satisfied, the processing in the “if statement” is still performed, but when the “if-else statement” is used, the processing in the “else statement” is performed if the conditional expression is not satisfied.

Note that the else is not indented; it is in the same position as the “if statemet”.
if-elif-else statement
Apart from simply deciding between two outcomes based on whether a particular condition is met or not, you can also create more complex scenarios with three or more branches. This means considering various conditions and their combinations.
Let’s illustrate this with a practical Python example. Imagine you have a program that evaluates students’ scores and provides different remarks based on their performance. Instead of just having “Pass” and “Fail” as options, you might want to include an “Excellent” category for scores of 90 or higher.
In this case, you’d define three conditions:
- “Pass” if the score is 60 or higher.
- “Fail” if the score is less than 60.
- “Excellent” if the score is 90 or higher.
This way, depending on the student’s score, the program can branch into three distinct outcomes, offering more nuanced feedback.

One example of how this can be accomplished is by creating an if inside an else.
score = int(input("Please enter the score: "))
if score >= 90:
print("Excellent!")
else:
if score >= 60:
print("Pass!")
else:
print("Fail!")
print("DONE")
Initially, the code checks whether the score is greater than or equal to 90. If the score is 90 or above, it proceeds to execute the corresponding code block(“Excellent”). However, if the score is not 90 or above, it then evaluates whether the score is 60 or above, constituting the condition specified in the “else statement”. Depending on the fulfillment of this condition, the program will output either “Pass” or “Fail.”
When score = 97 :
Excellent!
DONE
When score = 73 :
Pass!
DONE
When score = 30 :
Fail!
DONE
Another method is to append the clause “elif” between if and else.
score = int(input("Please enter the score: "))
if score >= 90:
print("Excellent!")
elif score >= 60:
print("Pass!")
else:
print("Fail!")
print("DONE")
This Python script takes input for a score, evaluates it, and then outputs a message based on the specified conditions. The messages vary for scores above 90, between 60 and 89, and below 60. Finally, a concluding message is printed indicating the completion of the process.
The result will be the same as above.
“elif” can be used for multipule conditions.
score = int(input("Please enter the score: "))
if score >= 90:
print("Excellent!")
elif score >= 80:
print("Very Good!")
elif score >= 60:
print("Pass!")
elif score >= 40:
print("Needs Improvement.")
else:
print("Fail!")
print("DONE")
When score = 95 :
Excellent!
DONE
When score = 81 :
Very Good!
DONE
When score = 63 :
Pass!
DONE
When scoe = 45 :
Need Improvement!
DONE
When score = 25 :
Fail!
DONE
If the number of branches is large, use elif.
Assignment 1: Calculate the total price including shipping
Specifications:
- Display “Please enter the pre-tax price:” and accept input for the pre-tax price from the keyboard.
- Upon entering the pre-tax price, calculate the 10% tax-inclusive price, rounding down to the nearest whole number.
- If the calculated tax-inclusive price is $20 or more, display “Free shipping.” However, if it’s less than $20, indicate “There is a $3.5 shipping fee” and add $3.5 to the price.
- Display the total price, including shipping, on the screen in the format “The total price including shipping is $XXX.”
This is one example code:
# Display "Please enter the pre-tax price:" and receive the pre-tax price input from the keyboard
tax_exclusive_price = float(input("Please enter the pre-tax price: $"))
# Calculate the tax-inclusive price, rounding down to the nearest whole number
tax_inclusive_price = int(tax_exclusive_price * 1.1)
# Calculate the shipping cost
shipping_cost = 0.0
if tax_inclusive_price >= 20:
print("Free shipping!")
else:
print("Shipping cost: $3.5")
shipping_cost = 3.5
# Calculate the total price including shipping
total_price = tax_inclusive_price + shipping_cost
# Display the result
print(f"The total price including shipping is ${total_price}.")
This program, when the user inputs the pre-tax price, calculates the tax-inclusive price and shipping cost, and then displays the final price.
Explanation:
- Save the pre-tax price entered from the keyboard in the variable “tax_exclusive_price”. “float(input(…))” prompts the user to input a numerical value and converts it to a floating-point number.
- Calculate the tax-inclusive price by applying a 10% tax rate to the pre-tax price and use “int()” to round down to the nearest whole number.
- Set the initial value of “shipping_cost” to 0.0. If the tax-inclusive price is $20 or more, shipping is free. Otherwise, there is a $3.5 shipping cost.
- Save the final price, including tax and shipping, in the variable “total_price”.
- Display the result on the screen.
Repetition
Now you will learn how to repeat the same process.
For example, suppose you want to display the integers 1 through 10 consecutively.
It is easier to write,
“Repeat the same process only while satisfying a specific conditional expression,”
or
“Repeat the same process only 10 times.”
To make such statements, use the “while” and for loops.
while statement
First, let’s learn about the “while statement”, which repeats a process “for as long as a certain conditional expression is satisfied.

i = 0
while i < 5:
i += 1
print(i)
print("DONE")
Output :
1
2
3
4
5
DONE
When using the “while statement” in Python, the syntax is quite similar to the “”if statement””. The “while statement” allows you to repeatedly execute a block of code as long as a specified condition is true. Here’s another example:
# Example: Print numbers 0 to 4 using a while loop
counter = 0
# The loop continues as long as the counter is less than 5
while counter < 5:
print(counter)
counter += 1 # Increment the counter by 1 in each iteration
Output :
0
1
2
3
4
In this example, the “while statement” checks the condition “counter < 5”. As long as this condition is true, the indented block of code inside the “while statement” will be executed. The program prints the current value of the “counter” and then increments it by 1. This process repeats until the condition becomes false.
It’s important to note that the “while statement” itself does not inherently keep track of the number of iterations. To count the iterations, you need to declare a variable (in this case, “counter”) before the “while statement”. This variable serves as a counter, storing the number of repeats. By initializing the counter before the loop and incrementing it within the loop, you can effectively keep track of the number of iterations.
In summary, the “while statement” allows you to repeat a set of instructions while a condition is true. To keep track of the number of repetitions, a counter variable is used and incremented during each iteration. This counter variable is commonly referred to as an index or a loop variable.
Infinite Loops
In the given code snippet, the while loop has a conditional expression “i >= 0”, which means the loop will continue executing as long as the value of variable “i” is greater than or equal to 0. The loop increments “i” by 1 in each iteration and prints its value. Here’s the code:
But don’t excute this code! It will go infinate loop!
i = 0
while i >= 0:
i += 1
print(i)
print("DONE")
However, due to the nature of the loop, where “i” is always incremented and the condition is always true (“i” will never become negative in this loop), the program will enter into an infinite loop. An infinite loop is a situation where the loop continues to execute endlessly, and the program never reaches the end.
It’s crucial to be cautious while implementing repetitive processes to avoid unintentional infinite loops. In the context of this example, the loop will keep printing incremented values of “i” indefinitely.
rewrite as follows;
i = 0
while i < 10: # output: number from 1 to 10
i += 1
print(i)
print("DONE")
If you find yourself in an infinite loop situation during program execution, or if you have mistakenly run a program similar to the one above, you can stop the process as follows:
- Locate the toolbar at the top of the Jupyter Notebook.
- Look for the square-shaped “■” (stop) button. This button is used to interrupt the execution of the current cell or kernel.
- Click on the “■” button. This action will interrupt the kernel and stop the execution of the code.
After interrupting the kernel, you may need to restart it if the notebook is unresponsive. You can do this by selecting “Kernel” from the menu and choosing “Restart & Clear Output” or “Restart & Run All” depending on your needs.
Keep in mind that interrupting the kernel forcefully stops the execution of all code cells, and any unsaved progress will be lost. If you have important data that you don’t want to lose, make sure to save your Jupyter Notebook regularly.
Break
The “break” statement is used in Python to exit a loop prematurely. It is particularly useful when dealing with infinite loops created intentionally with a “while True:” construct. Here’s an example illustrating the use of “break”:
i = 0
while True:
i += 1
if i > 5:
break
print(i)
print("DONE")
In this example, the “while True:” creates an infinite loop because the condition is always true. To prevent an infinite loop, the “break” statement is used within the loop. The loop will continue executing until “i” exceeds 5, at which point the “break” statement is triggered, and the loop is terminated.

The program then prints the current value of “i” within the loop. However, when the “break” statement is executed, any code below it within the same loop scope will not be executed.
Therefore, the “print(“DONE”)” statement is outside the loop and will only be executed after the loop terminates.
In the end, this code will print numbers from 1 to 5 and then print “DONE” to indicate that the loop has been terminated. The use of “break” allows you to control the flow of your loops based on specific conditions, providing a way to exit the loop prematurely.
Continue
In Python, the “continue” statement is used to skip the rest of the code inside a loop for the current iteration and return to the beginning of the loop for the next iteration. It is particularly useful when you want to bypass certain conditions and continue the loop. Let’s explore its usage through an example:
i = 0
while True:
i += 1
if i > 10:
break
if i % 2 == 1:
continue
print(i)
print("DONE")
Output:
2
4
6
8
10
DONE
In this example, we have a “while True:” loop that increments the variable “i” in each iteration until “i” becomes greater than 10. Inside the loop, there’s an additional “if” statement checking if “i” is an odd number (“i % 2 == 1”). If this condition is true, the “continue” statement is executed.
When “continue” is encountered, the code skips the “print(i)” statement and returns to the beginning of the loop. Therefore, only even numbers from 1 to 10 will be printed on the screen, as the odd numbers trigger the “continue” statement, bypassing the “print(i)” line.

This demonstrates how “continue” can be used to control the flow of the loop, skipping specific iterations based on certain conditions. In this case, it allows us to print only even numbers within the specified range.
for loop
The “for loop” in Python provides another way to implement repetitive processes, complementing the “while loop”. It is particularly useful when the number of iterations is known in advance. Unlike “while”, which is more flexible when the number of iterations changes dynamically, “for” is suitable for situations where the number of iterations is fixed.
The primary difference between “for” and “while” is in their syntax. Despite this difference, both are used for iterative processes, and you can apply “break” and “continue” statements with either.
Now, let’s explore a practical example using the “for loop”:
for i in range(6):
print(i)
Output:
0
1
2
3
4
5
The indented block of code under the “for loop” is the body of the loop, and it is executed for each value of “i”.
- “range(6)” generates a sequence of numbers from 0 to 5.
- “for i in …” assigns each value from the sequence to the variable “i” one by one.
- The indented block of code under the “for” statement is the body of the loop, and it is executed for each value of “i”.
With just a few lines of code, we can display consecutive integer values. Although the syntax might initially seem a bit unfamiliar, understanding the breakdown of “for i in range(6):” makes it more intelligible.
More information about range() function
Example 1: Using “range()” for Iterating Over User Inputs
for _ in range(3): # Repeat 3 times
user_input = input("Enter something: ")
print(f"You entered: {user_input}")
In this example, the loop asks the user to input something three times and prints each input.
Example 2: Summing Numbers in a Range
total_sum = 0
for num in range(1, 6): # Sum numbers from 1 to 5
total_sum += num
print(f"The sum of numbers from 1 to 5 is: {total_sum}")
This example demonstrates using a “for” loop to calculate the sum of numbers from 1 to 5.
Example 3: Printing Even Numbers Using a Step
for even_num in range(2, 11, 2): # Print even numbers from 2 to 10
print(even_num)
Here, the loop prints even numbers from 2 to 10 using a step of 2.
Example 4: Counting Down and Skipping Odd Numbers
for i in range(10, 0, -2): # Count down by 2, skipping odd numbers
print(i)
This example counts down from 10 to 2, skipping odd numbers.
Example 5: Multiplication Table
for i in range(1, 6):
for j in range(1, 6):
print(f"{i} x {j} = {i * j}")
In this practical example, the nested loop generates a multiplication table from 1 to 5.
Remember, these examples are meant to show practical use cases of “for loop” in Python. Feel free to experiment with them and adapt them to your own projects!
Assignment 2: Calculate Class Average
Write a Python program to calculate the average score of a class of students.
Requirements:
- Create an infinite loop using a “while” statement.
- Inside the loop, prompt the user to enter the test score (an integer value). Store the entered score in a variable.
- Keep track of the total score by adding each entered score to a running total variable.
- Count the number of students who have entered their scores using a counter variable.
- If the entered score is -1, end the iteration and proceed to calculate the average score.
- Calculate the average score using the formula: “total score / number of students.”
- Display the result: “The average score of XX students for the test is △△△ points.”
Example:
Enter the score (or -1 to finish): 60
Enter the score (or -1 to finish): 70
Enter the score (or -1 to finish): 80
Enter the score (or -1 to finish): 90
Enter the score (or -1 to finish): 100
Enter the score (or -1 to finish): -1
5 students' average test score is 80.0 points.
Here’s a sample code to solve the problem of calculating the average score for a class of students:
# Initialize variables
total_score = 0
student_count = 0
while True: # Infinite loop
score_input = input("Enter the score (or -1 to finish): ")
if score_input == "-1":
break
score = int(score_input)
# Add score to the total
total_score += score
student_count += 1
# Calculate average score
if student_count > 0:
average_score = total_score / student_count
print(f"{student_count} students' average test score is {average_score:.1f} points.")
else:
print("No scores entered.")
This code uses a “while True” loop to continuously prompt the user for test scores until -1 is entered. It validates the input to ensure it’s a valid integer and accumulates the total score and counts the number of students. Finally, it calculates and displays the average score when the loop ends.
Runtime Errors (Exceptions) and Exception Handling
The following is an additional challenge to the previous assignment. What if a user enters a non-integer value, can you prompt them to enter the score that are valid integers.
Exception Handling
There are two main types of “errors”:
- syntax errors
- runtime errors (exceptions).
“Syntax errors” are errors caused by incorrectly written Python code, and the Cloud9 editor will indicate the incorrect line with a red cross.
On the other hand, “runtime errors (exceptions)” are errors that may occur due to the state of variables or external files during processing. Since they are “possible” and do not occur in all cases, the Cloud9 editor cannot catch them as “errors.
In the provided code:
score_input = input("Please enter the score (or -1 to finish): ")
If someone accidentally types anything other than a number, like “abc,” it will result in an error:
ValueError: invalid literal for int() with base 10: 'abc'
This happens because the “int()” function, used to convert data to integer values, cannot process data with non-numeric characters, leading to a runtime error (exception). When an exception occurs, the program stops running, and any instructions written below it won’t be executed.
To prevent the program from crashing when an exception occurs, we can use “try” and “except“.
In the code, when we use a “try” statement, it’s like telling the program to try doing something, and if it faces a problem (an exception), we have a plan (using “except”) to handle that problem without crashing the whole program.
For example, let’s say we want the user to enter a number, but if they accidentally type something that’s not a number, the program might have trouble. Here’s how we handle that:
try:
score = int(score_input)
except ValueError:
print("Oops! That's not a valid number. Please enter a valid number.")
In this code:
- The “try” block attempts to get user input and convert it to an integer.
- If everything goes smoothly, it continues with the code inside the “try” block.
- But, if the user enters something that cannot be converted to a number (like a word or symbol), a “ValueError” occurs.
- Instead of crashing the program, we gracefully handle this situation by jumping to the “except” block. Inside the “except” block, we print a friendly error message to guide the user.
So, in simple terms, the “try” and “except” blocks help the program to keep going even if something unexpected happens, and we have a backup plan to handle those unexpected situations.
# Initialize variables
total_score = 0
student_count = 0
while True: # Infinite loop
score_input = input("Please enter the score (or -1 to finish): ")
if score_input == "-1":
break
# Validate the input as an integer
try:
score = int(score_input)
except ValueError:
print("Oops! That's not a valid number. Please enter a valid number.")
continue # Skip the rest of the loop and ask for input again
# Add score to the total
total_score += score
student_count += 1
# Calculate average score
if student_count > 0:
average_score = total_score / student_count
print(f"{student_count} students' average test score is {average_score:.1f} points.")
else:
print("No scores entered.")
When “abc” was entered:
Oops! That's not a valid number. Please enter a valid number.
In the “try:” clause, you describe the process that may raise an exception. In this case, we want to deal with “an exception when anything besides number is applied to int(),” so we put the first line of the instruction in the try: clause. As with conditional branches and iterations, indentation is a valid range for try, so be sure not to forget it.
By specifying “except exception name:”, you can define what to do when the specified exception occurs. The exception that may occur here is ValueError, so we have written except ValueError:. In this case, we simply display a message like “Oops! That’s not a valid number. Please enter a valid number.”
Here is another type of error.
try:
numerator = int(input("Enter the numerator: "))
denominator = int(input("Enter the denominator: "))
result = numerator / denominator
print(f"The result of the division is: {result}")
except ZeroDivisionError:
print("Error: Cannot divide by zero!")
except ValueError:
print("Error: Please enter valid numbers.")
In this example:
- The “try” block attempts to perform a division operation (“numerator / denominator”).
- If the user enters a denominator of zero, a “ZeroDivisionError” occurs.
- The “except ZeroDivisionError” block handles this specific error and prints an error message.
- If the user enters a non-numeric value, a “ValueError” occurs.
- The “except ValueError” block handles this specific error and prints another error message.
Assignment 03: Handling Division Errors
Write a Python program to perform division. Prompt the user to enter the numerator and denominator. Handle potential errors like zero division and invalid input using “try-except”.
Requirements:
- Use “try” and “except” to handle potential errors during division.
- If the denominator is zero, print an error message stating “Cannot divide by zero!”
- If the user enters a non-numeric value, print an error message stating “Please enter valid numbers.”
- Display the result of the division if there are no errors.
Example Output:
Enter the numerator: 8
Enter the denominator: 4
The result of the division is: 2.0
Enter the numerator: 7
Enter the denominator: 0
Error: Cannot divide by zero!
Enter the numerator: car
Enter the denominator: 6
Error: Please enter valid numbers.
sample code:
# Handling Division Errors
try:
# Get user input for numerator and denominator
numerator = int(input("Enter the numerator: "))
denominator = int(input("Enter the denominator: "))
# Perform division
result = numerator / denominator
# Display the result
print(f"The result of the division is: {result}")
except ZeroDivisionError:
print("Error: Cannot divide by zero!")
except ValueError:
print("Error: Please enter valid numbers.")
Summary
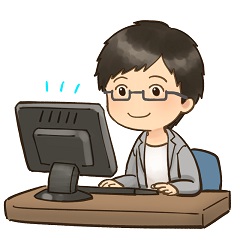
This article introduces important programming concepts like “conditional branching,” “iterations,” and “exception handling.” Unlike basic sequential processing, these concepts empower you to make decisions, repeat actions, and manage errors in your code. By understanding these fundamentals, you’ll be well-equipped to create more dynamic and advanced programs!