In Python, everything is treated as an object, whether it’s a number, a word, a list, or even a function.
What Is Attributes and Methods in Python
Intuitively, these objects behave like real objects. This means that each piece of data, like numbers or text, has its own set of attributes (like properties) and methods (like actions).
To understand the nature of objects, imagine “books” as an example.

attributes (properties): A book has attributes such as “title,” “author,” “year of publication,” and “number of pages”. These attribute information are known as “properties”.
methods (actions). Books “open,” “close,” and “turn pages”. A “method” represents the behavior of an object.
There are many other characteristics of objects, but at this stage it is important to understand that they have attributes and methods.
And objects have relationships to each other. For example, a book is created by its author. Therefore, there is a “created” relationship between the objects “book” and “author”.
For example, let’s look at some examples:
# Define an object with attributes
book = {
"title": "python for beginners",
"year": 2024,
"author": "Tom Smith"
}
# Accessing attributes of the object
print(book["title"])
print(book["year"])
# Example of an object with method
print(book["author"].upper()) # Method: convert the string to uppercase
Output:
python for beginners
2024
TOM SMITH
Difference Between a Method and a Function
A method of an object corresponds to an instruction that can be executed in Python. A function is also an instruction. So what is the difference between a “function” and a “method”?
A “function” such as print() or input() was written as follows
Function name (argument)
In contrast, a “method” is described as follows
object.Method name (arguments)
The difference is the presence or absence of the dot (.). Functions are provided by Python, so you could simply specify only the function name. However, a method is an object’s property, so it must be written in the form of “execute object’s method”.
String Methods
Strings in Python come with a variety of built-in methods that can be used to perform different operations on strings. Let’s explore some basic methods.
Converting Letters to Uppercase and Lowercase
The lower()
method converts all letters in a string to lowercase, while the upper()
method converts all letters to uppercase. Here’s an example demonstrating how these methods can be used:
text = "Hello World"
print(text.lower()) # Convert all letters to lowercase
print(text.upper()) # Convert all letters to uppercase
print(text)
Output:
hello world
HELLO WORLD
Hello World
It’s important to note that calling these methods does not modify the original string (text
). This characteristic is known as a non-destructive method.
To modify the original string:
text = "Hello World"
text = text.lower() # Convert all letters to lowercase and modify the original string
print(text)
text = text.upper() # Convert from lowercase to uppercase and modify the orinal string
print(text)
Output:
hello world
HELLO WORLD
In the above code, the original string text
is first converted to lowercase and stored in the variable text
. Then, the text
is further converted to uppercase and stored in the text
again. At the end, the string text
is a uppercase.
Finding a String

To locate a specific character or substring within a string, you can utilize the find()
method.
text = "Hello World"
index = text.find("l")
print(index) # Output: 2
Output:
2
In this example, the find()
method searches for the letter “l” within the string “Hello World” and returns its index. If the character is not found, it returns -1
.
String Replacement
When you need to substitute one substring with another within a string, the replace()
method comes in handy.
text = "It is a sunny day."
replaced_text = text.replace("sunny", "rainy")
print(replaced_text)
Output:
It is a rainy day.
The replace()
method replaces occurrences of a specified substring with another string.
Trimming of Strings

To eliminate leading and trailing whitespace from a string, use the strip()
method.
text = " Hello World "
trimmed_text = text.strip()
print(trimmed_text)
Output:
Hello World
This operation removes any extraneous whitespace from the beginning and end of the string.
Embedding Variables in a String
In Python, you can include the value of a variable directly within a string using either the format()
method or f-strings.
How to Use the format() Method
name = "Tom"
age = 25
message = "My name is {} and I am {} years old.".format(name, age)
print(message)
Output:
My name is Tom and I am 25 years old.
The format()
method inserts the values provided as arguments into {}
placeholders within the string, in the specified order.
How to Use f-strings
name = "Tom"
age = 25
message = f"My name is {name} and I am {age} years old."
print(message)
Output:
My name is Tom and I am 25 years old.
f-strings allow you to directly embed the value of a variable by prefixing the string with f
and placing the variable name within {}
.
By utilizing these methods, you can effortlessly incorporate variable values into strings.
Summary
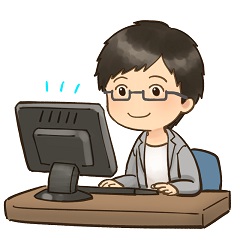
This article explains basic concept of attributes and methods in python. And for Python beginners, I show basic string methods, including string searching, replacement, trimming, and embedding variable values. There are numerous methods available, and mastering them can greatly enhance your ability to program in Python
.